Android
Connecting to Evernote with Android Intents

Android was designed to make it easy to build apps that work together, so it's a great platform for developing apps that work with Evernote. There are two basic ways for your Android app to work with Evernote: through the Evernote web service API, and using intents.
Getting Started
To get started, visit the Cloud API page and download the Evernote API ZIP file. The file contains our API client library as well as sample code that demonstrates the use of the API and intents on Android.
Using the Evernote API
Your Android application can communicate directly with the Evernote web service ("the cloud") using our API. Using the API gives you complete access to a user's Evernote account. You can create new notes, search, read and update existing notes, and generally perform any operation that our own client apps perform. Users don't need to have Evernote for Android installed in order for you to use the API.
To use the Evernote API on Android, your application will need to import our API client Java library and the Apache Thrift runtime, lib/java/evernote-api-[version].jar
and lib/java/libthrift.jar
. In addition, you should use the Android-specific code in src/android
, which is more memory efficient than the default Thrift code. You'll find sample code that demonstrates the use of the API in a simple Android app in our API ZIP file under sample/android/HelloEDAM
.
Using Intents
Evernote for Android supports a number of intents that your application can send to invoke various actions, such as creating a new note with an image attached. Integrating with Evernote for Android can be easier than working with our API, because we take care of the networking, error handling, etc. However, these actions will only work if the user has Evernote for Android version 2.0 or later installed on their device, has launched it at least once and has signed into their Evernote account.
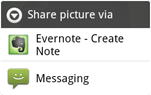
To see how this works, install Evernote for Android on your device, then open your image gallery. Choose an image, then tap the menu button and tap "Share". You should see "Evernote - Create Note" in the list of apps that you can share the image with. Choose Evernote and you'll be taken to our "new note" UI, where your photo has been added as an attachment.
The table below lists the intents that your application can send to Evernote for Android.
You can find sample code that demonstrates the use of each intent in our API ZIP file under sample/android/HelloEvernote
.
Action | Data | Type | Result |
---|---|---|---|
SEND | application/* image/* audio/* video/* text/* |
The standard Android SEND action can be used to send files to Evernote for Android. You specify the Uri of the file that you want to send using the EXTRA_STREAM field. You can specify a title for the new note using the EXTRA_TITLE or EXTRA_SUBJECT field. When invoked, Evernote will display the "new note" UI with the specified file as an attachment. The function "shareImage" in our Android sample code demonstrates the use of this intent. |
|
text/plain |
To create a new note with pre-populated content (instead of a file attachment), add the desired text to the EXTRA_TEXT field. You can specify a title for the new note using the EXTRA_TITLE or EXTRA_SUBJECT field. When invoked, Evernote will display the "new note" UI with the specified text in the note body. The function "newNoteWithContent" in our Android sample code demonstrates the use of this intent. |
||
file://path | application/enex |
If you want to take full control over the contents of a note, you can create a file formatted using Evernote's export file format, which is documented in the Evernote Export DTD: https://xml.evernote.com/pub/evernote-export.dtd. When invoked, Evernote for Android will verify that the file is correctly formatted and queue your new note for upload. No user interface is displayed. The function "createNoteUsingEnex" in our Android sample code demonstrates the use of this intent. |
|
SEND_MULTIPLE | application/* image/* audio/* video/* text/* |
You can use the standard Android SEND_MULTIPLE action to send multiple files to Evernote for Android. You specify the Uris of the files that you want to send using the EXTRA_STREAM field. You can specify a title for the new note using the EXTRA_TITLE or EXTRA_SUBJECT field. |
|
com.evernote.action.CREATE_NEW_NOTE |
This action gives you the most control over passing information to Evernote for Android's New Note activity. When invoked, Evernote for Android will display the "new note" UI as though the user had tapped the "new note" button in the Evernote widget. You can specify a title for the new note using the EXTRA_TITLE field. You can specify plaintext content for the new note using the EXTRA_TEXT field. You can specify the notebook for the new note by passing the NOTEBOOK_GUID extra field, which takes a String notebook GUID. The notebook must already exist. You can specify tags for the new note by passing the TAG_NAME_LIST extra field, which takes an ArrayList of String tag names. If a tag does not exist, it will be created. You can specify the Note's author attribute by passing a String in the AUTHOR extra field. You can specify the Note's sourceURL attribute by passing a String in the SOURCE_URL extra field. You can specify the Note's sourceApplication attribute by passing a String in the SOURCE_APP extra field. You can attach one or more files to the new note by specifying the Uris of the files using the EXTRA_STREAM field. The functions "newNoteWithContent" and "newNoteWithContentAndAttachment" in our Android sample code demonstrate the use of this intent. This intent is available in Evernote for Android 3.0 and later. |
||
com.evernote.action.SEARCH_NOTES |
The SEARCH_NOTES action can be used to run a query within Evernote for Android. You specify the query string using the QUERY. When invoked, Evernote for Android will display the Evernote note list UI containing a list of notes that match the query that you passed. If you do not pass a query, all notes will be shown. The function "doSearch" in our Android sample code demonstrates the use of this intent. This intent is available in Evernote for Android 2.5.1 and later. |
||
com.evernote.action.VIEW_NOTE |
The VIEW_NOTE action can be used to display a single note in Evernote for Android. You specify the note to display by passing its GUID in the NOTE_GUID extra field. When invoked, Evernote for Android will display the Evernote note with the specified GUID. If there is no note with that GUID, the note viewer will display the first note in the user's current note list. You can specify that the note viewer be displayed without the Evernote title bar at the top of the screen by passing the FULL_SCREEN extra field with the value "true". The function "viewNote" in our Android sample code demonstrates the use of this intent. This intent is available in Evernote for Android 2.5.1 and later. |
||
com.evernote.action.NEW_SNAPSHOT |
When invoked, Evernote for Android will display the camera UI as though the user had tapped the "new photo note" button in the Evernote widget. You cannot pass any additional information using this intent. |
||
com.evernote.action.NEW_VOICE_NOTE |
When invoked, Evernote for Android will display an empty "new note" UI with the audio recorder started as though the user had tapped the "new voice note" button in the Evernote widget. You cannot pass any additional information using this intent. |
||
com.evernote.action.SEARCH |
When invoked, Evernote for Android will display the Evernote search UI as though the user had tapped the "search" button in the Evernote widget. You cannot pass any additional information using this intent. |