App Notebooks
Connecting your application to a single notebook
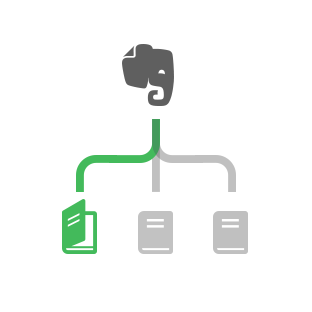
This is a new feature of the Evernote API!
For more information about this new feature for the Evernote API, read our announcement on our developer blog: [POST] Feedback for App Notebooks
Overview
Historically, authorized Evernote API keys have had access to all notes and notebooks in a given user’s account. Moving forward, the Evernote API creation process will give developers the option to limit their app’s access to a single notebook in the user’s account. We’re calling this feature App Notebook. It will provide greater security of user data as well as straightforward implementation for developers.
This document will describe how developers should implement this feature, as well as the user flow.
Implementation
By and large, the process of authenticating with the Evernote API will remain the same as with legacy API keys, with a handful of differences.
When an authentication token is issued for an API key configured for App Notebook:
- Calls to
NoteStore.listNotebooks
will return a collection containing only the single notebook to which the API key has access. - Calls to
NoteStore
functions that attempt to access or modify notebooks other than the app’s notebook will result in aEDAMUserException
with thePERMISSION_DENIED
error code.
To include existing linked or business notebooks as options from which the user can choose their destination notebook, include supportLinkedSandbox=true
in the OAuth URL during the authentication process:
https://www.evernote.com/OAuth.action?oauth_token=[...]&preferRegistration=true&
supportLinkedSandbox=true
When a user selects a shared or business notebook as the notebook for use with your application, the OAuth callback will have the sandbox_lnb
parameter set to true
. Otherwise the sandbox_lnb
is set to false
by default
Error Handling
It is possible that the user may delete the notebook to which your application has access. In such a case, NoteStore.listNotebooks
and NoteStore.listLinkedNotebooks
will return empty result sets and NoteStore.getNotebook
will result in an EDAMNotFoundException
.
Your application should be prepared to handle this situation. Our recommendation is to alert the user as to what happened and prompt them to re-authorize your application, allowing them to select a different notebook.
try {
NoteStore.getNotebook(notebookGuid);
} catch EDAMNotFoundException {
// Rerun authentication process so the user can choose a new notebook
// Then get the GUID of the new notebook via listNotebooks
notebooks = NoteStore.listNotebooks()
if notebooks isn’t empty:
newGuid = notebooks[0].guid
NoteStore.getNotebook(newGuid)
// etc.
}
As with legacy authentication to an entire user’s account, third-party developers should also build their application to elegantly handle the situation in which the authentication token expires or is revoked by the user. See the “Introduction to OAuth” section of our Authentication documentation for more information.
User Flow
As with the OAuth process, the user authentication flow will remain largely the same. Historically, the authentication screen notified the user that a given application would have access to their account for one year and which specific permissions the application would have (create notes, update notes, etc.). The primary difference now is that users will be presented with the option to choose the notebook used by the application requesting authorization. By default, a new notebook will be created in the user’s account called [application name].
Suggesting a Notebook Name
To suggest a notebook name to the user, add supportLinkedSandbox
and suggestedNotebookName
to your OAuth parameters. Set supportLinkedSandbox
to true
and suggestedNotebookName
to the URL encoded name you would like to suggest to the user. For example:
https://www.evernote.com/OAuth.action?oauth_token=[...]&
supportLinkedSandbox=true
&suggestedNotebookName=Suggested%20Notebook%20Name
where "Suggested Notebook Name" is the URL encoded name you would like to suggest to the user. If the user already has a notebook in their account with the name you suggest, that notebook will be selected. If there is no notebook in the user's account with the name you suggest through OAuth, a notebook with the suggested name will be created in the users account. Suggested notebook names must follow the same restrictions as standard Evernote notebook names as outlined in in the API reference documentation here.
The user can choose to select the change notebook option in the OAuth flow for selecting any existing notebooks.
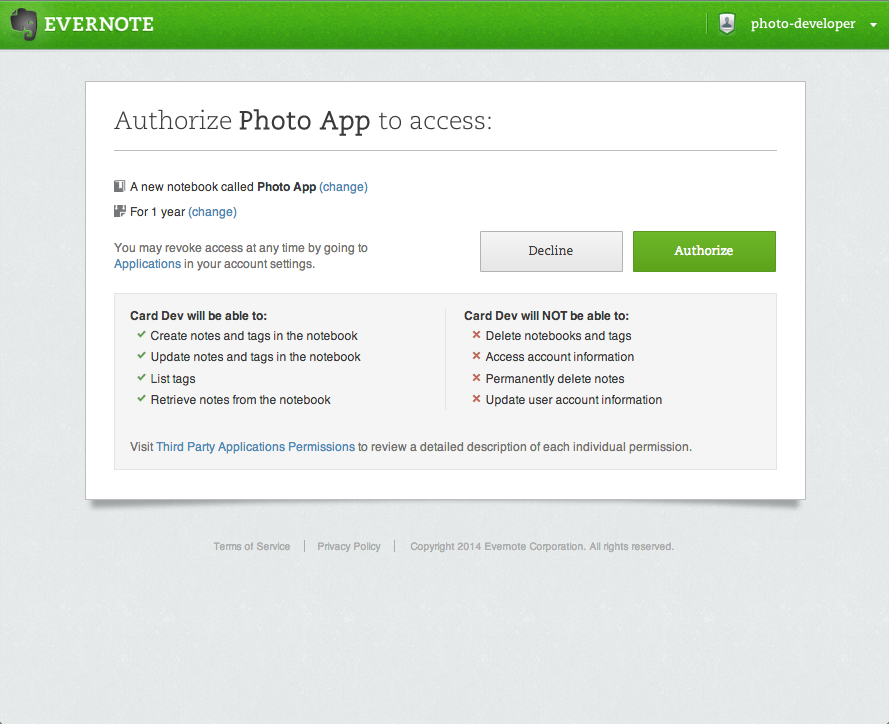
During the Authentication flow, users will have the option to change the default notebook:
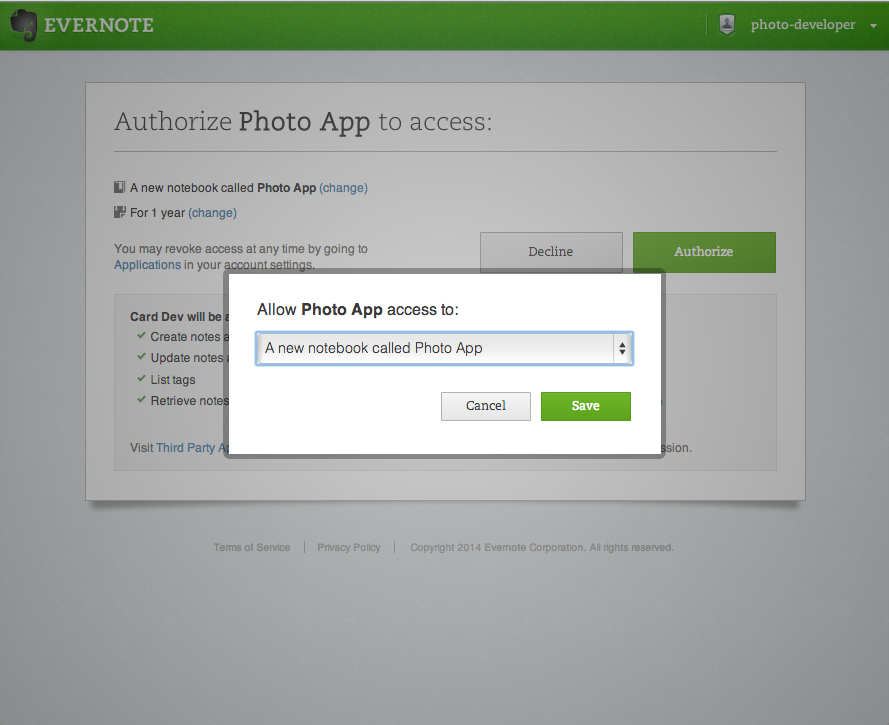
The selected notebook can be from a user's personal, shared, and business notebooks:
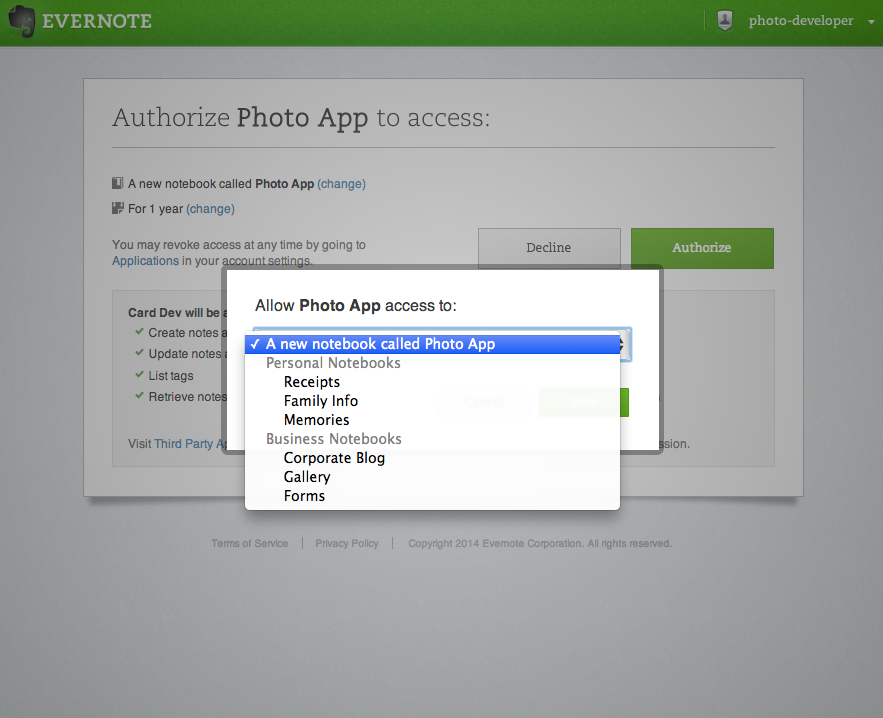